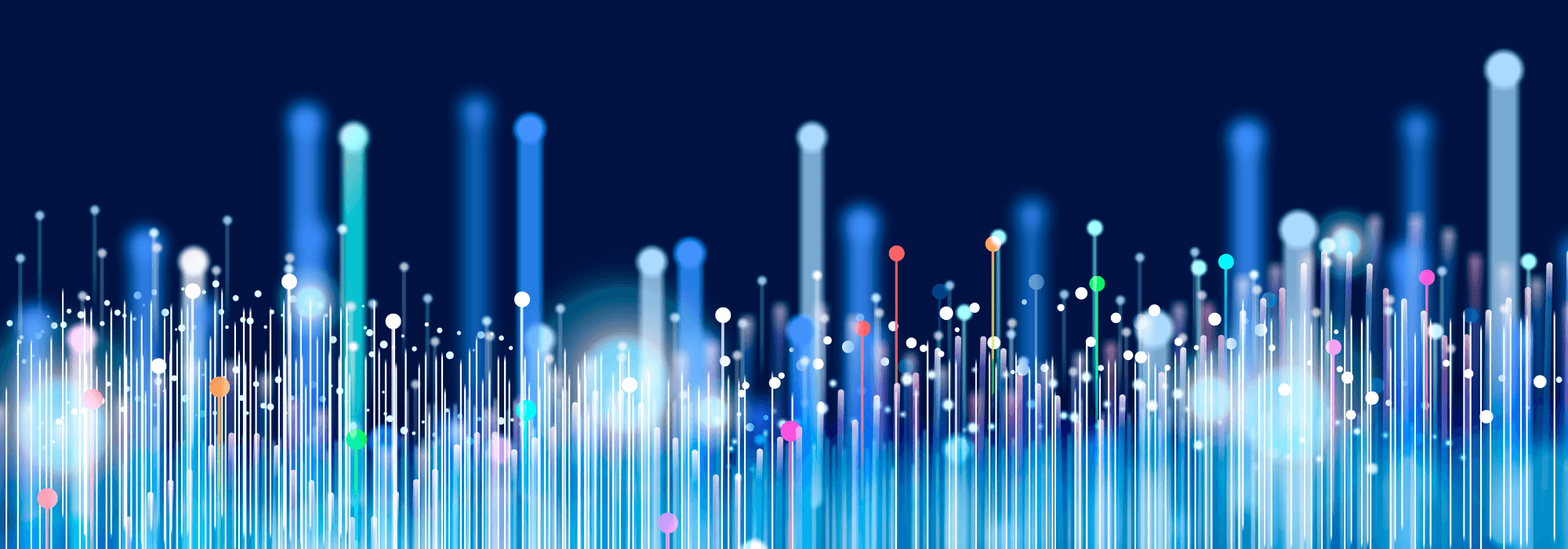
Reasons React is popular?
PJ LeBlanc / September 6, 2024
Reasons to Use React JS in 2024
Strong Community Support
One of the cornerstones of React JS’s success is its vibrant and active community. The React community is one of the largest in the web development world, with numerous forums, GitHub repositories, and dedicated user groups. This extensive network provides developers with ample resources, from troubleshooting tips to advanced tutorials, ensuring that help is always at hand.
Ecosystem
React JS boasts a comprehensive ecosystem that integrates seamlessly with a variety of tools and libraries. Whether you need state management (Redux, MobX), routing (React Router), or styling (Styled Components, Emotion), there’s a tool for every requirement. This rich ecosystem simplifies development processes and enhances productivity.
Virtual DOM
React’s introduction of the Virtual DOM revolutionised how web applications handle updates. By using a Virtual DOM, React ensures that only the parts of the DOM that need to change are updated, significantly boosting performance compared to traditional DOM manipulation methods.
Component-Based Architecture
React makes it easy to create reusable UI components. The component-based architecture promotes modularity and reusability, which drives down app development costs. Developers can create self-contained components that manage their state and behaviour, making maintaining and scaling applications easier. This approach reduces redundancy and enhances code maintainability.
Official Documentation
React’s official documentation is renowned for its clarity and comprehensiveness. It covers everything from basic concepts to advanced techniques, making it accessible for developers of all skill levels.
State management
State management is a crucial aspect of building robust and scalable applications in React. As applications grow in complexity, managing the state becomes increasingly challenging. To address this, different state management approaches have emerged, offering developers various tools and methodologies. Three popular categories in the React ecosystem are Centralized, Reactive, and Atomic state management.
'use client';
import { useState } from 'react';
import { Button } from './ui/button';
import { MinusIcon, PlusIcon } from '@radix-ui/react-icons';
export default function Counter() {
const [count, setCount] = useState(0);
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
return (
<div className='flex items-center gap-3'>
<Button onClick={decrement} variant="outline" size='icon'>
<MinusIcon />
</Button>
<span>{count}</span>
<Button onClick={increment} variant="outline" size='icon'>
<PlusIcon />
</Button>
</div>
);
}